What is a Library Crate?
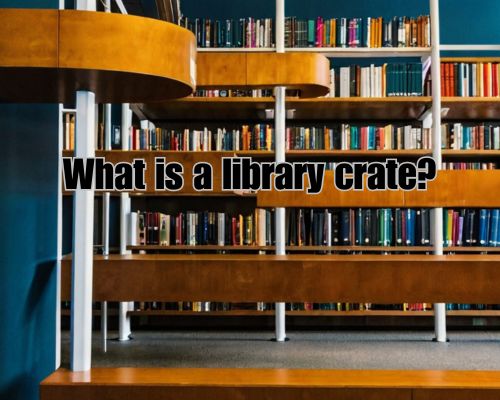
Understanding Its Role in Software Development
In Rust programming, understanding the concept of a library crate is essential for managing and sharing code effectively.
“A library crate is a type of crate in Rust that compiles to a shared library, as opposed to an executable. This means it doesn’t have a main()
function. Instead, it typically contains reusable functions, types, and modules that can be called and used in other projects.” said Leona Rodriguesi Founder Of Mornington Cabinet Makers.
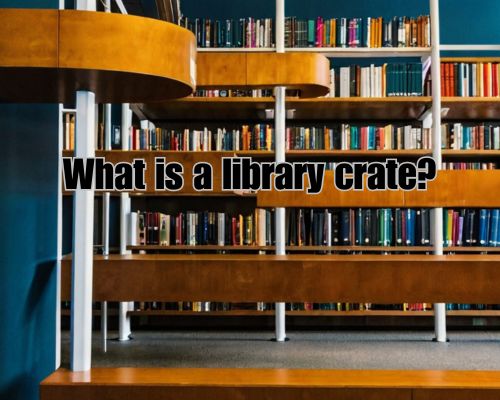
When you create a package in Rust, it can contain multiple binary crates, but only one library crate.
A library crate usually starts with a lib.rs
file, where you define the core functionality that you intend to reuse across different parts of your application or even in completely different projects.
This crate can then be shared via Cargo, Rust’s package manager, making it an integral part of the Rust ecosystem.
The modular nature of Rust allows you to organise your code into various modules within the library crate, providing clear separation and scope control.
Leveraging library crates can significantly enhance the maintainability and scalability of your Rust programs, enabling you to build more complex and robust applications over time.
Understanding Library Crates in Rust
Library crates are a fundamental component in Rust, facilitating code reuse, modular design, and easier maintenance. In this section, we’ll explore what library crates are, how to create them, and best practices for their structure and organisation.
Definition and Purpose
A library crate in Rust is a package that compiles into a library rather than an executable. It provides reusable code, which can be shared across multiple projects.
The main purpose of a library crate is to offer functionality organised into modules. These modules can then be used by other developers through a well-defined public interface. This supports efficient code reuse and helps manage dependencies with ease.
Library crates are distinguished by the presence of a src/lib.rs
file, which serves as the entry point. This contrasts with executable crates that have a src/main.rs
file.
By designing code as libraries, you create modular, maintainable systems that facilitate collaboration and evolution over time.
Creating a Library Crate
To create a library crate, start with the cargo new
command:
cargo new my_library --lib
The --lib
flag instructs Cargo to generate a library crate. This creates a directory containing a src
folder with a lib.rs
file. The lib.rs
file is the crate root and the starting point for your library’s source code.
In the lib.rs
file, you define the public interface of your library using the pub
keyword. For example:
pub mod my_module {
pub fn my_function() {
// Function implementation
}
}
Publishing your library crate can be done via crates.io, making it available for others to use.
Simply define your crate’s metadata in the Cargo.toml
file, including name, version, and authorship, then execute the publication command:
cargo publish
This process makes your library accessible for other developers to add as dependencies in their projects.
Structuring and Organising Code
Effective code organisation within a library crate is achieved through Rust’s module system. You structure your code in logical modules by creating files and directories, thereby maintain clean, readable, and maintainable codebases.
For example, you might split implementations into different files:
src/
├── lib.rs
├── module1.rs
└── module2.rs
In your lib.rs
file, include these modules using mod
:
mod module1;
mod module2;
To expose functions, structs, or enums to the users of your library, use the pub
keyword. Scope management is crucial to ensure users only have access to what they need.
Thanks to Rust’s rich documentation capabilities, you can generate documentation comments using triple slashes (///
). This creates comprehensive and user-friendly doc pages.
Careful design of your library crate ensures that all necessary symbols and functionalities are accessible, while keeping your internal code paths clean and manageable.
Compiling and Managing Library Crates
“When working with library crates in Rust, it’s essential to understand the compilation process, package management through Cargo, and the importance of versioning and publishing. These aspects ensure your library crate is reliable, maintainable, and easily distributable.” said Leona Rodriguesi Founder Of Mornington Cabinet Makers.
Compilation and the Rust Compiler
The Rust compiler (rustc
) is responsible for converting your source code into binaries.
For a library crate, you typically start with a lib.rs
file. Use the cargo build
command to compile your library crate.
This command reads from the Cargo.toml
file, which contains metadata and dependencies.
For efficient development, Rust compiles to the target/debug/
directory by default.
You can also use cargo test
to run unit and integration tests, ensuring your library functions as expected.
Consider dev-dependencies
in Cargo.toml
for tools necessary only during development and testing.
Package Management with Cargo
Cargo is Rust’s package manager and build system. It manages dependencies, compiles your code, runs tests, and more.
Your Cargo.toml
file is crucial, defining your crate’s dependencies in sections like [dependencies]
and [dev-dependencies]
.
With cargo
, you can organise your projects into workspaces, allowing multiple related projects to share a common set of dependencies and code.
To fetch and manage dependencies from crates.io, use cargo update
to ensure you’re using the latest versions specified in your Cargo.toml
.
Versioning and Publishing
Versioning your library crate ensures users can rely on stable APIs.
Semantic versioning (semver
) is commonly used in Rust. It guides you to increment version numbers based on changes.
Update the version in Cargo.toml
as you release new features or fix bugs.
To publish a library crate to crates.io, first create an account. Then, obtain an API token from the account settings page.
Add this token with cargo login
.
Use cargo publish
to upload your crate. This makes it available to the wider Rust community.
Proper versioning and detailed documentation assist users in integrating your library effectively.